
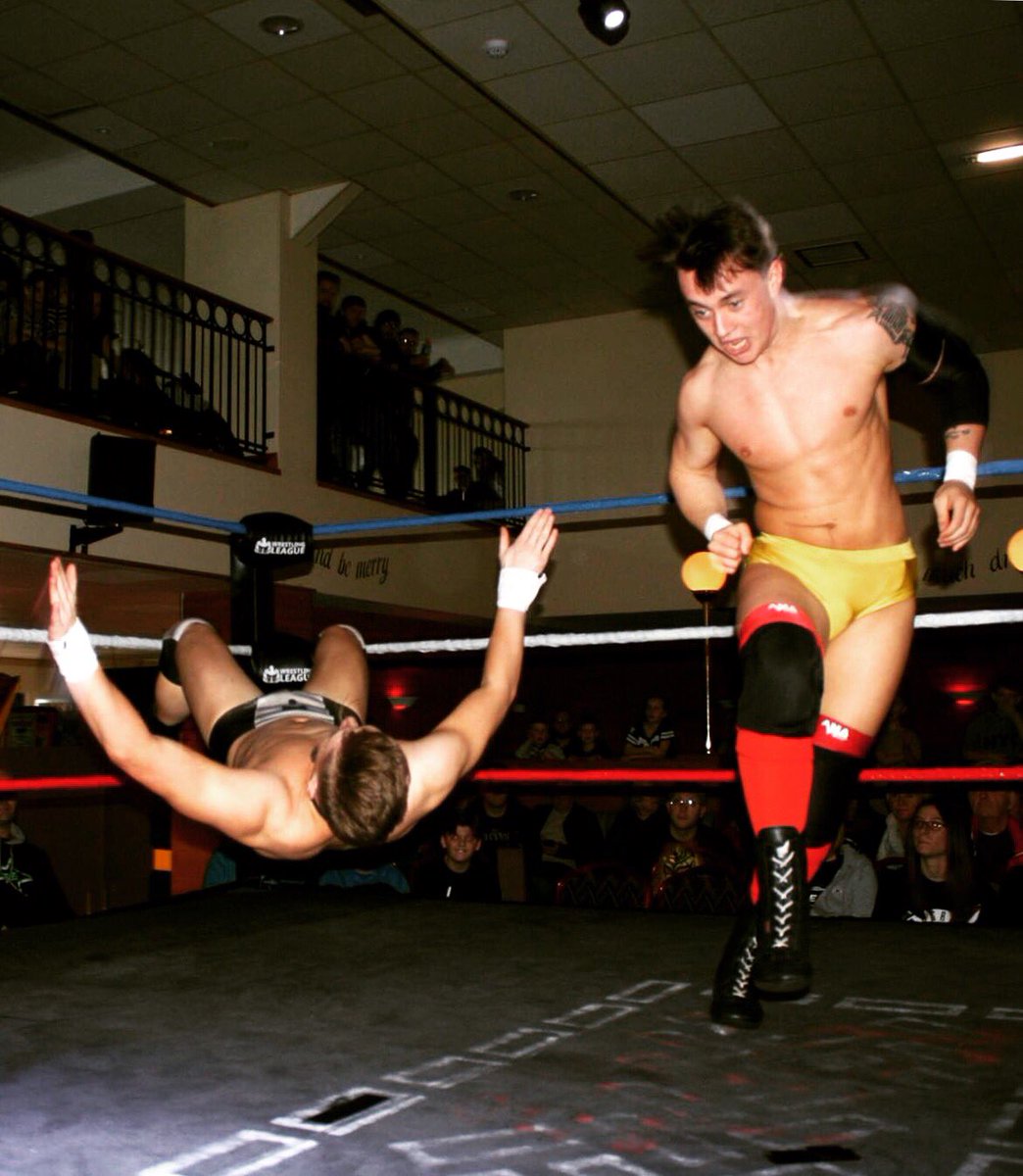
Hence, parseURL("") will result in a Success containing the created URL, whereas parseURL("garbage") will result in a Failure containing a MalformedURLException.

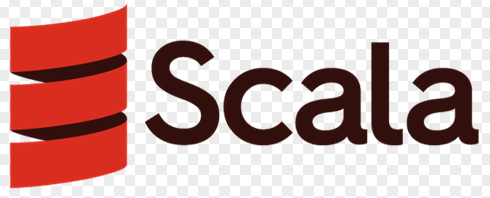
Inside that method, non-fatal exceptions are caught, returning a Failure containing the respective exception. For our example, this means that the new URL(url) is executed inside the apply method of the Try object. This method expects a by-name parameter of type A (here, URL). To achieve this, we are using the apply factory method on the Try companion object. If the URL constructor throws a MalformedURLException, however, it will be a Failure. If the given url is syntactically correct, this will be a Success. One part of our application will be a function that parses the entered URL and creates a from it: import ĭef parseURL(url: String): Try = Try(new URL(url))Īs you can see, we return a value of type Try. The user will be able to enter the URL of the web page they want to fetch. This makes the possibility explicit and forces clients of our function to deal with the possibility of an error in some way.įor example, let's assume we want to write a simple web page fetcher. If we know that a computation may result in an error, we can simply use Try as the return type of our function. If, on the other hand, it represents a computation in which an error has occurred, it is an instance of Failure, wrapping a Throwable, i.e. There are two different types of Try: If an instance of Try represents a successful computation, it is an instance of Success, simply wrapping a value of type A. Instances of such a container type for possible errors can easily be passed around between concurrently executing parts of your application. Where Option is a container for a value of type A that may be present or not, Try represents a computation that may result in a value of type A, if it is successful, or in some Throwable if something has gone wrong.

The semantics of Try are best explained by comparing them to those of the Option type that was the topic of the previous part of this series. There is also a similar type, called Either, which, even after the introduction of Try, can still be very useful, but is more general. In this article, we are confining ourselves to the Try type that was introduced in Scala 2.10 and later backported to Scala 2.9.3. Rather, in Scala, we are using a specific type that represents computations that may result in an exception. Hence, in Scala, it's usually preferred to signify that an error has occurred by returning an appropriate value from your function.ĭon't worry, we are not going back to C-style error handling, using error codes that we need to check for by convention. For instance, if you need to deal with an exception thrown by an Actor that is executed on some other thread, you obviously cannot do that by catching that exception – you will want a possibility to receive a message denoting the error condition. It's also a rather bad solution for applications with a lot of concurrency.
#Scala try catch code#
Now, having this kind of exception handling code all over your code base can become ugly very quickly and doesn't really go well with functional programming. Like these languages, Scala allows you to throw an exception: case class Customer(age: Int)Ĭase class UnderAgeException(message: String) extends Exception(message)ĭef bu圜igarettes(customer: Customer): Cigarettes = Throwing and catching exceptionsīefore going straight to Scala's idiomatic approach at error handling, let's first have a look at an approach that is more akin to how you are used to working with error conditions if you come from languages like Java or Ruby. I'm using features introduced with Scala 2.10 and ported back to Scala 2.9.3, so make sure your Scala version in SBT is at 2.9.3 or later. In this article, I'm going to present Scala's approach to dealing with errors, based on the Try type, and the rationale behind it. Scala, as it turns out, is pretty well positioned when it comes to dealing with error conditions in an elegant way. The importance of how well a language supports you in doing so is often underestimated, for some reason or another. As soon you want to create anything serious, though, you can no longer run away from handling errors and exceptions in your code. When just playing around with a new language, you might get away with simply ignoring the fact that something might go wrong.
